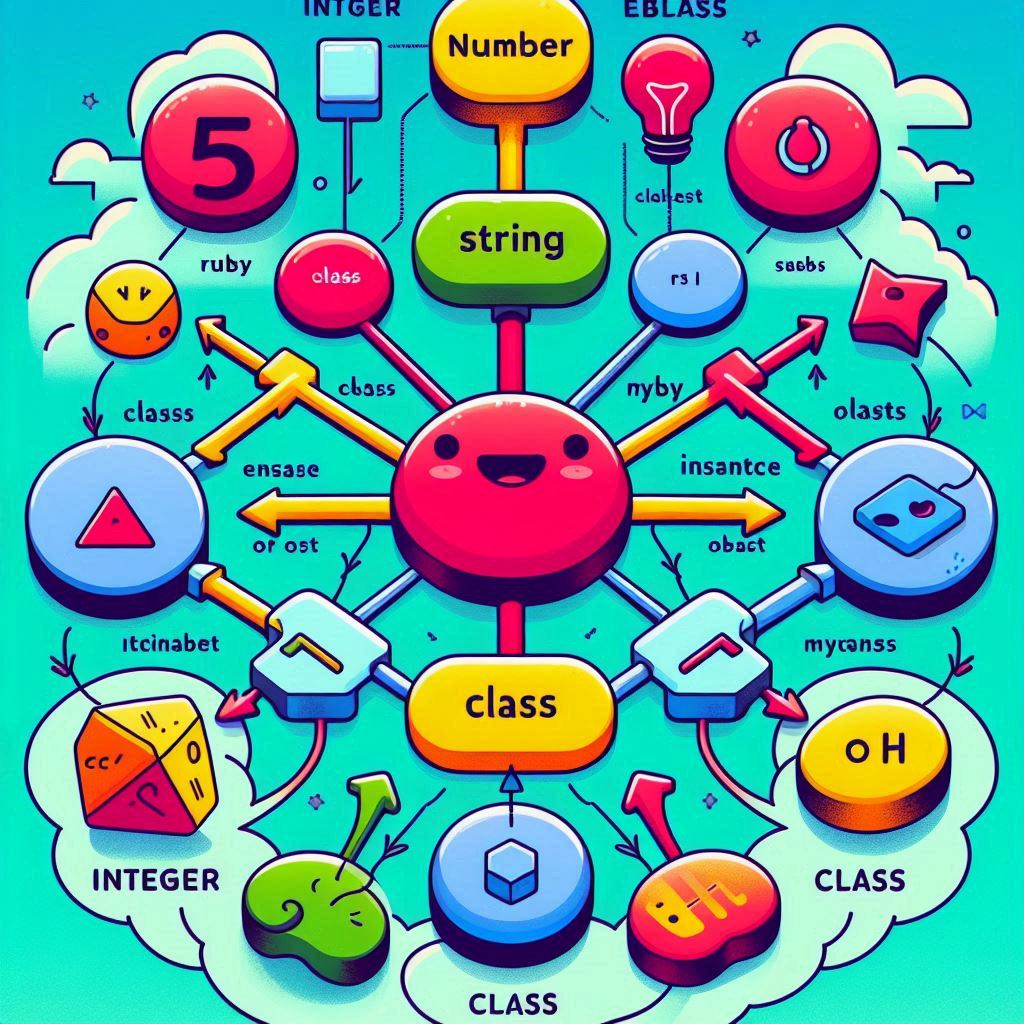
Everything is an Object in Ruby: Even Classes
Ruby Ruby on Rails Software CraftsmanshipRuby is a pure object-oriented programming language, and one of the key features that sets it apart from many other languages is its philosophy that everything is an object. Whether you’re working with strings, numbers, arrays, or even classes themselves, everything in Ruby is treated as an object. This is a powerful concept that simplifies the language and makes it more consistent and flexible. Let’s dive deeper into what this means, especially the idea that even classes are objects in Ruby.
Objects in Ruby: The Foundation
In Ruby, objects are the building blocks of your code. Almost everything you manipulate is an object. For instance:
# Numbers are objects
5.class # => Integer
# Strings are objects
"hello".class # => String
As you can see, the .class method returns the class of the object, confirming that numbers and strings are instances of their respective classes. This makes sense—after all, an integer 5
is an instance of the Integer class, and "hello"
is an instance of the String
class.
Classes Are Objects Too
But here’s where it gets really interesting: even classes are objects in Ruby.
In Ruby, a class is an instance of the class Class
. This means that classes themselves are objects, and you can treat them like any other object—call methods on them, pass them around as arguments, and even create them dynamically.
Let’s see this in action:
# Define a simple class
class MyClass
end
# Check the class of MyClass
MyClass.class # => Class
So, MyClass
is an object, and it belongs to the class Class
. This demonstrates that classes are first-class citizens in Ruby.
The Relationship Between Objects and Classes
At first glance, this can seem circular. If classes are instances of the class Class
, and classes define the objects, how does this work under the hood?
Here’s the basic hierarchy:
Every object in Ruby is an instance of some class.
Each class is itself an instance of the class Class
.
The Class
class is a subclass of Module, which allows it to include methods and constants.
We can illustrate this further with code:
# Integer is a class
Integer.class # => Class
# Class itself is an instance of Class
Class.class # => Class
Even Class is an object! It’s mind-bending but elegant: everything in Ruby stems from Class, which itself is an object, thereby making the system highly flexible and consistent.
Metaclasses: Classes for Single Objects
In Ruby, every object can have its own class called a metaclass (or singleton class). This is a hidden class that Ruby creates behind the scenes to hold methods that are unique to a specific object.
For instance:
str = "hello"
# Define a singleton method just for this instance of the string
def str.shout
self.upcase + "!"
end
str.shout # => "HELLO!"
In this case, the shout method only exists on the str
object. The str
object has a metaclass that holds this method. If you try calling shout on a different string, it won’t work:
another_str = "world"
another_str.shout # => NoMethodError: undefined method `shout'
Ruby achieves this dynamic flexibility by creating a metaclass for each object, allowing objects to behave in ways that are customized and extendable at runtime.
Creating Classes Dynamically
Since classes are objects in Ruby, you can create classes dynamically. You can define new classes at runtime using Class.new
.
Here’s an example:
MyDynamicClass = Class.new do
def greet
"Hello, world!"
end
end
obj = MyDynamicClass.new
obj.greet # => "Hello, world!"
This illustrates how flexible Ruby is—classes can be defined and modified dynamically, allowing for powerful metaprogramming techniques.
Conclusion
Ruby’s “everything is an object” philosophy is more than just a slogan; it’s a core feature of the language that makes it both flexible and consistent. By treating even classes as objects, Ruby opens the door to dynamic class creation, powerful metaprogramming, and a more intuitive approach to object-oriented programming.
Understanding that everything in Ruby is an object, including classes themselves, can help you unlock the full potential of the language and write more dynamic, flexible code. It’s one of the reasons Ruby is loved by so many developers, especially those who value elegance and simplicity in software design.
Next time you write a Ruby class, remember: you’re not just defining a blueprint for objects; you’re working with an object itself!