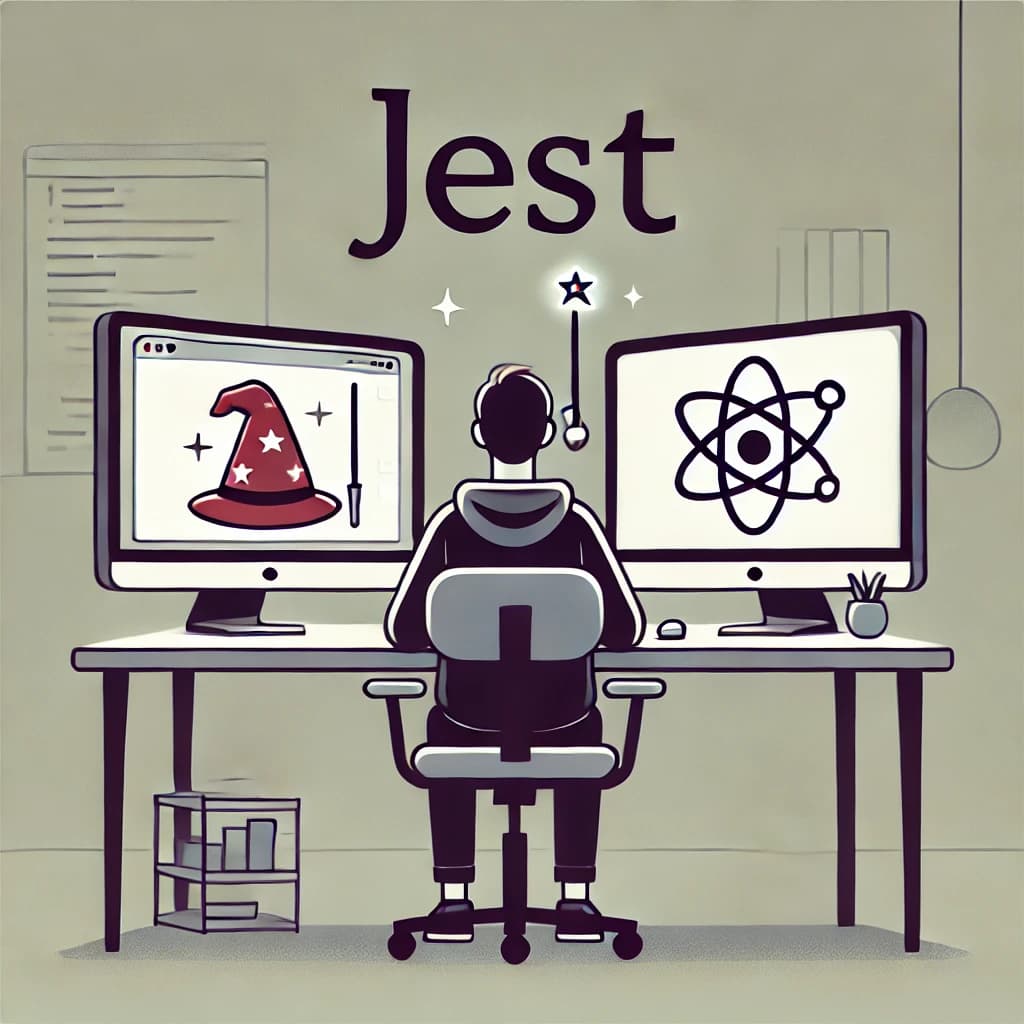
Exploring Different Types of Matchers in Jest for React Testing
React Jest TestingTesting is a crucial part of modern software development, and Jest, combined with React Testing Library, has become a popular choice for testing React applications. One of Jest’s most powerful features is its extensive collection of matchers, which allow you to make assertions about your code in a clear and concise manner. In this blog, we’ll explore the various types of matchers available in Jest and how they can be leveraged to write robust tests for React applications.
What Are Jest Matchers?
In Jest, matchers are methods that enable you to compare the actual output of your code with the expected outcome. These matchers are chained to expect
statements, providing a readable way to express test conditions.
Here’s a quick example:
expect(component.textContent).toBe('Hello, World!');
In this case, toBe
is a matcher that verifies that the actual value exactly matches the expected value.
Common Categories of Jest Matchers
1. Basic Matchers
Basic matchers are used to compare primitive values, such as strings, numbers, or booleans.
Examples:
expect(2 + 2).toBe(4); // Strict equality
expect(component.title).toEqual('My Title'); // Deep equality for objects or arrays
expect(array).not.toBeUndefined(); // Negating conditions
2. Truthiness Matchers
These matchers are used to check if a value is truthy or falsy.
Examples:
expect(value).toBeNull();
expect(value).toBeDefined();
expect(value).toBeTruthy();
expect(value).toBeFalsy();
3. Number Matchers
When working with numbers, you can use specific matchers to assert ranges or comparisons.
Examples:
expect(value).toBeGreaterThan(10);
expect(value).toBeGreaterThanOrEqual(10);
expect(value).toBeLessThan(20);
expect(value).toBeCloseTo(0.3, 5); // For floating-point numbers
4. String Matchers
String matchers make it easy to verify string values and patterns.
Examples:
expect(text).toMatch(/React/); // Regex matching
expect(text).toContain('Welcome'); // Substring containment
5. Array and Iterable Matchers
Jest also provides matchers to test arrays and iterables.
Examples:
expect(shoppingList).toContain('Milk');
expect(users).toHaveLength(3);
expect(set).toContainEqual({id: 1, name: 'John'});
6. Object Matchers
For object comparison, Jest has matchers that allow you to assert the presence or absence of properties.
Examples:
expect(user).toHaveProperty('name');
expect(user).toHaveProperty('age', 30);
expect(user).toMatchObject({name: 'Alice'});
7. Mock Function Matchers
When testing React components or hooks, mock functions are often used to simulate behaviors or track interactions.
Examples:
const mockCallback = jest.fn();
mockCallback('arg1', 'arg2');
expect(mockCallback).toHaveBeenCalled();
expect(mockCallback).toHaveBeenCalledWith('arg1', 'arg2');
expect(mockCallback).toHaveBeenCalledTimes(1);
8. Asynchronous Matchers
React applications often involve asynchronous operations, such as API calls. Jest provides matchers to handle promises.
Examples:
await expect(fetchData()).resolves.toEqual({data: 'Success'});
await expect(fetchData()).rejects.toThrow('Error');
Using Matchers with React Testing Library
React Testing Library is commonly used with Jest to test React components. Matchers help verify DOM elements, user interactions, and component states.
Examples:
import {render, screen} from '@testing-library/react';
import userEvent from '@testing-library/user-event';
import MyComponent from './MyComponent';
// Render the component
render(<MyComponent />);
// Check if an element exists
expect(screen.getByText('Hello, React!')).toBeInTheDocument();
// Simulate a user action
userEvent.click(screen.getByRole('button'));
expect(screen.getByText('Clicked!')).toBeVisible();
The @testing-library/jest-dom
library extends Jest with custom matchers like toBeInTheDocument
and toBeVisible
, making tests more intuitive.
Writing Effective Tests with Matchers
To write effective tests:
- Be Specific: Use the most precise matcher for the condition you’re testing.
- Combine Matchers: Utilize
.not
for negative cases and.resolves
or.rejects
for async tests. - Keep It Readable: Write assertions that clearly express the intent of your test.
Conclusion
Jest’s extensive library of matchers makes it a powerful tool for testing React applications. From simple equality checks to complex object comparisons and asynchronous operations, these matchers provide everything you need to ensure your components behave as expected. By mastering these matchers and combining them with React Testing Library, you can create tests that are both reliable and maintainable.
Happy testing!